字典是 Python 中的映射数据类型,工作原理类似 Perl 中的关联数组或者哈希表,由键-值(key-value)对构成。几乎所有类型的 Python 对象都可以用作键,不过一般还是以数字或者
字符串最为常用。
值可以是任意类型的 Python 对象,字典元素用大括号({ })包裹
通过键而不是下标来访问元素值
In [33]: dict={'host':'earth'}
In [34]: dict['port']=80
In [35]: dict
Out[35]: {'host': 'earth', 'port': 80}
In [33]: dict={'host':'earth'}
In [34]: dict['port']=80
In [35]: dict
Out[35]: {'host': 'earth', 'port': 80}
In [36]: dict.keys()
Out[36]: ['host', 'port']
In [37]: dict['host']
Out[37]: 'earth'
In [38]: for key in dict:
....: print key,dict[key]
....:
host earth
port 80
创建字典:
>>> dict1={}
>>> dict2={'name':'earth','port':80}
>>> dict1,dict2
({}, {'name': 'earth', 'port': 80})
>>> fdict=dict((['x',1],['y',2]))
>>> fdict
{'y': 2, 'x': 1}
>>> ddict={}.fromkeys(('x','y'),-1)
>>> ddoct
Traceback (most recent call last):
File "<stdin>",line 1, in <module>
NameError: name 'ddoct' is not defined
>>> ddict
{'y': -1, 'x': -1}
如何访问字典中的值:
>>> dict2
{'name': 'earth', 'port': 80}
>>> for key in dict2.keys():
... print 'key=%s,value=%s'%(key,dict2[key])
...
key=name,value=earth
key=port,value=80
>>> print dict2.keys()
['name', 'port']
>>> for key in dict2:
... print 'key=%s,value=%s'%(key,dict2[key])
...
key=name,value=earth
key=port,value=80
>>> for key in dict2:
... print 'key=%s,value=%s'%(key,dict2[key])
...
key=name,value=earth
key=port,value=80
>>> dict2
{'name': 'earth', 'port': 80}
>>> dict2['name']
'earth'
>>> dict2['port']
80
>>> print 'host %s is running on port %d'%(dict2['name'],dict2['port'])
host earth is running on port 80
查询一个键是否在字典中:
>>> 'server' in dict2
False
>>> 'name' in dict2
True
>>> for key in dict3:
... print'key=%s,value=%s' %(key,dict3[key])
...
key=1,value=3.14
key=1,value=ABC
key=3.2,value=xyz
更新字典
>>> dict2
{'name': 'earth', 'port': 80}
>>> dict2['name']='venus'
>>> dict2
{'name': 'venus', 'port': 80}
>>> dict2['arch']='sunos5'
>>> dict2
{'arch': 'sunos5', 'name': 'venus', 'port': 80}
>>> print 'host %(name)s is running on port %(port)d' %dict2
host venus is running on port 80
删除字典元素和字典
del dict2['name'] # 删除键为“name”的条目
dict2.clear() # 删除 dict2 中所有的条目
del dict2 # 删除整个 dict2 字典
dict2.pop('name') # 删除并返回键为“name”的条目
对序列类型来说,用索引做唯一参数或下标(subscript)以获取一个序列中某个元素的值。
对字典类型来说,是用键(key)查询(字典中的元素),所以键是参数(argument), 而不是一个索引
(index)。键查找操作符既可以用于给字典赋值,也可以用于从字典中取值:
dict()
>>> dict([('xy'[i-1],i) for i in range(1,3)])
{'y': 2, 'x': 1}
len()
对字典
调用 len(),它会返回所有元素(键-值对)的数目:
>>> dict9
{'host': 'earth', 'port': 80}
>>> len(dict9)
2
hash()
内建函数 hash()本身并不是为字典设计的方法,但它可以判断某个对象是否可以做一个字典的键
只有这个对象是可哈希的,
才可作为字典的键 (函数的返回值是整数,不产生错误或异常)。
映射类型内建方法
>>> mydict={'host':'earch','port':80}
>>> mydict
{'host': 'earch', 'port': 80}
>>> mydict.keys()
['host', 'port']
>>> mydict.keys()
['host', 'port']
>>> mydict.items()
[('host', 'earch'), ('port', 80)]
>>> mydict.setdefault('prot','tcp')
'tcp'
>>> mydict
{'host': 'earch', 'prot': 'tcp', 'port': 80}
>>> {}.fromkeys(('love','honor'),True)
{'love': True, 'honor': True}
>>> dict1
{'a': 10, 'b': 20}
>>> dict1.has_key('a')
True
>>> dict1.get('a')
10
字典的键
不允许一个键对应多个值
你必须明确一条原则:每个键只能对应一个项
键必须是可哈希的
passwd.py
#!/usr/bin/env python
db={}
def newuser():
prompt = 'login desired:'
while True:
name =raw_input(prompt)
if db.has_key(name):
prompt ='name taken,try another:'
continue
else:
break
pwd = raw_input('passwd:')
db[name]=pwd
def olduser():
name = raw_input('login:')
pwd = raw_input('passwd:')
passwd = db.get(name)
if passwd == pwd:
print 'welcomeback',name
else:
print 'loginincorrect'
def showmenu():
prompt="""
(N)ew User Login
(E)xisting User Login
(Q)uit
Enter choice:"""
done = False
while not done:
chosen = False
while not chosen:
try:
choice = raw_input('prompt').strip()[0].lower()
except(EOFError,KeyboardInterrupt):
choice = 'q'
print'\nYou picked:[%s]'% choice
if choicenot in 'neq':
print 'invalid option,try again'
else:
chosen = True
done= True
newuser()
olduser()
if __name__ == '__main__':
showmenu()
例子:
#!/usr/bin/env python
contacts={
'3951':['Alex','IT','SA'],
'4092':['Jack','HR','HR'],
'5122':['BlueTshirt','Sales','SecurityGuard']
}
print contacts['5122']
contacts['5122'][2]='Cleaner'
printcontacts['5122']

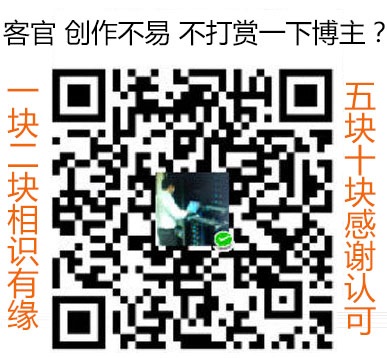
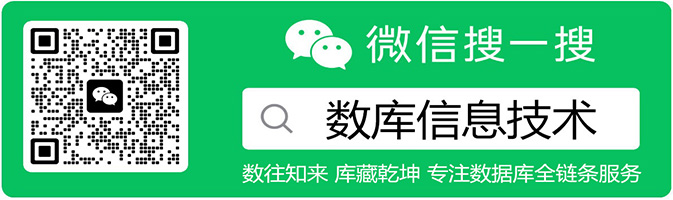